Python
"Python: Where simplicity meets power, and readability meets versatility."
- "Python: Web development, data science, automation, versatility, simplicity."
“Python: The elegant and versatile language for modern development. With its clean syntax and vast ecosystem, Python empowers developers to build anything from simple scripts to complex applications. From web development to data science, Python’s readability and simplicity make it a joy to learn and a powerhouse in the world of programming. Join the thriving community and unlock endless possibilities with Python today.”
(9.8k Learner)
python Program overview
python Certification Course
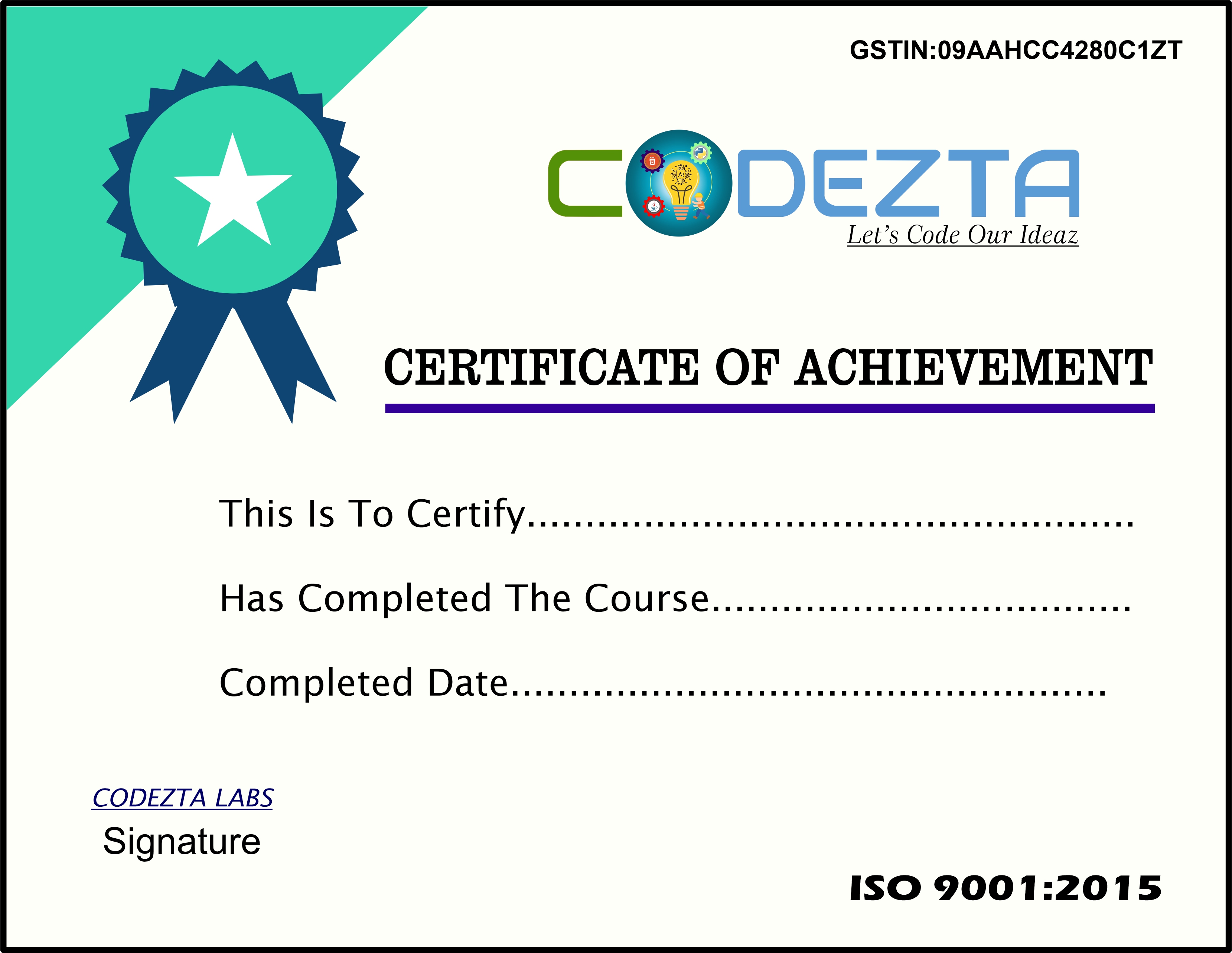
Key Features
Simple and Easy to Learn: Python’s syntax is clear and concise, making it easy for beginners to learn and understand.
Expressive Language: Python allows developers to express concepts with fewer lines of code compared to other languages, enhancing readability and maintainability.
Versatility: Python is a multipurpose language, suitable for web development, data science, artificial intelligence, automation, scripting, and more.
Large Standard Library: Python comes with a vast standard library that provides modules and functions for various tasks, reducing the need for external dependencies.
Community and Ecosystem: Python boasts a large and active community of developers who contribute to an extensive ecosystem of libraries, frameworks, and tools
Interpreted and Interactive: Python is an interpreted language, allowing for quick development cycles and interactive experimentation through tools like Jupyter Notebooks.
Platform Independence: Python code is portable and can run on different platforms without modification, thanks to its platform-independent nature.
Object-Oriented and Functional Programming: Python supports both object-oriented and functional programming paradigms, offering flexibility in coding styles.
Extensibility: Python can be easily extended by integrating modules written in other languages like C or C++, enhancing performance and functionality.
Strong Community Support: Python enjoys strong community support with regular updates, bug fixes, and enhancements, ensuring its relevance and reliability in modern software development.
What roles can a python programmer professional play?
Software Developer
Python programmers develop software applications, ranging from web applications and desktop GUIs to backend services and APIs. They use Python frameworks like Django, Flask, or PyQt to build scalable and efficient solutions.
Data Scientist/Analyst
Python is widely used in data science and analytics for tasks such as data cleaning, manipulation, visualization, and machine learning. Python programmers in this role work with libraries like NumPy, pandas, Matplotlib, and scikit-learn to analyze data and extract insights.
Machine Learning Engineer
Python is the primary language for machine learning and artificial intelligence. Python programmers in this role develop machine learning models, train them on large datasets, and deploy them into production environments using frameworks like TensorFlow or PyTorch.
DevOps Engineer
Python is commonly used for automation and scripting in DevOps workflows. Python programmers in this role write scripts to automate deployment processes, configure infrastructure using tools like Ansible or Terraform, and monitor system health using frameworks like Nagios or Prometheus
Game Developer
Python can be used for game development, particularly for scripting and prototyping. Python programmers in this role use libraries like Pygame or Panda3D to develop 2D and 3D games, and may also use frameworks like Unity or Unreal Engine that support Python scripting.
Cybersecurity Analyst
Python is used in cybersecurity for tasks such as network scanning, vulnerability assessment, and penetration testing. Python programmers in this role develop scripts and tools to automate security tasks and analyze security-related data.
Skills to Master
SQL
Python
Excel
Data Analysis
Data Mining
Tools to master
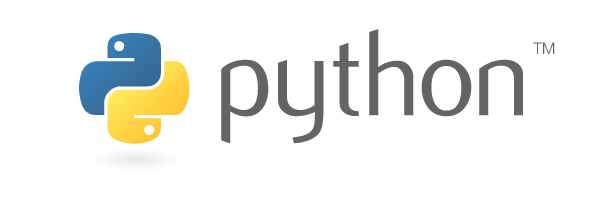
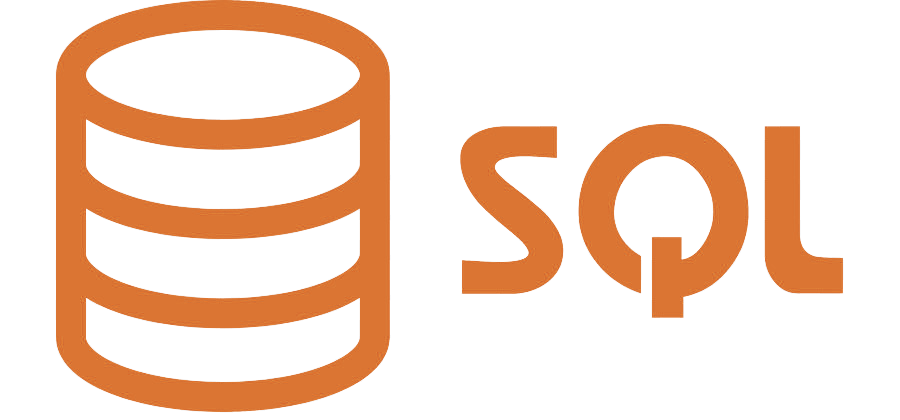

Course Content
Great! Let’s dive into Python lesson 1. In this lesson, we’ll cover the basics of Python programming, including variables, data types, and basic operations.
Variables and Data Types
In Python, you can store data in variables. Variables are like containers that hold different types of data. Here are some common data types in Python:
- Integers: Whole numbers without any decimal point.
- Floats: Numbers with decimal points.
- Strings: Text enclosed in single or double quotes.
- Booleans: True or False values.
- Lists: Ordered collection of items.
- Tuples: Similar to lists but immutable (cannot be changed).
- Dictionaries: Collection of key-value pairs.
Basic Operations
Python supports various operations on different data types:
- Arithmetic Operators: Addition (+), Subtraction (-), Multiplication (*), Division (/), Modulus (%), Exponentiation (**).
- Comparison Operators: Equal to (==), Not equal to (!=), Greater than (>), Less than (<), Greater than or equal to (>=), Less than or equal to (<=).
- Logical Operators: and, or, not.
- Assignment Operators: =, +=, -=, *=, /=, %=, **=.
- Membership Operators: in, not in.
In Python lesson 2, let’s delve deeper into control flow statements and functions.
Control Flow Statements
Control flow statements allow you to control the flow of your program’s execution based on certain conditions. The main control flow statements in Python are:
- Conditional Statements (if, elif, else): Allows you to execute certain code blocks based on conditions.
- Loops (for, while): Allows you to iterate over sequences or execute a block of code repeatedly.
Functions
Functions are blocks of reusable code that perform a specific task. They help in organizing your code and making it more modular. Functions have the following components:
- Function Definition: Defines the name of the function and its parameters.
- Function Body: Contains the code that performs the desired task.
- Return Statement: Specifies the value(s) that the function should return (optional).
In Python lesson 3, let’s cover more advanced topics including:
- Advanced Data Structures: We’ll explore sets and dictionaries.
- List Comprehensions: A concise way to create lists.
- Exception Handling: Dealing with errors gracefully
# Creating a set
my_set = {1, 2, 3, 4, 5}
# Adding elements to a set my_set.add(6)
# Removing elements from a set my_set.remove(3)
# Checking membership in a set print(2 in my_set) # Output: True
# Creating a dictionary
my_dict = {‘name’: ‘John’, ‘age’: 30, ‘city’: ‘New York’}
# Accessing values in a dictionary print(my_dict[‘name’])
# Output: John
# Adding a new key-value pair my_dict[‘gender’] = ‘Male’
# Removing a key-value pair del my_dict[‘age’]
In Python lesson 4, let’s explore more advanced concepts including:
- Object-Oriented Programming (OOP): Understanding classes and objects.
- Inheritance: Creating subclasses.
- Polymorphism: Using methods with the same name in different classes.
- Modules and Packages: Organizing code into reusable modules and packages.
Object-Oriented Programming (OOP)
OOP is a programming paradigm based on the concept of “objects”, which can contain data (attributes) and code (methods).
Projects
Projects will be a part of your Executive Post Graduate Certification Data Science & Artificial Intelligence to solidify your learning. They ensure you have real-world experience in Data Science and AI.
- Practice Essential Tools
- Designed by Industry Experts
- Get Real-world Experience
Machine Learning Models
Train machine learning models, such as regression or classification algorithms, to predict equipment failures or maintenance needs based on historical sensor data. Common techniques include linear regression, decision trees, random forests, and neural networks.
Maintenance Scheduling
Optimize maintenance schedules based on predicted failure probabilities and equipment usage patterns. This helps prioritize maintenance tasks and allocate resources efficiently.
Predictive Alerts
Generate predictive alerts or notifications when the system detects anomalies or predicts upcoming maintenance tasks. These alerts can be sent to maintenance technicians or managers via email, SMS, or a dashboard interface.